See the example:
System.Data.DataTable dt = obj.getInfoAsTable(); System.Data.DataView dv = dt.DefaultView(); dv.Sort = “[name], [dateOpt] DESC”;
This is an incorrect way to access the info because we are going back to the original info without the sorting:
Foreach(System.Data.DataRow r in dv.Table.Rows) { String name = r[“name”].toString(); }
This is the correct way of getting the sorted data:
Foreach(System.Data.DataRow r in dv.ToTable().Rows) { String name = r[“name”].toString(); }
The rows never physically get sorted. The view provides a sorted view of the data. The table never changes. You have to access the data through the view
to see it sorted. See the diagram below:
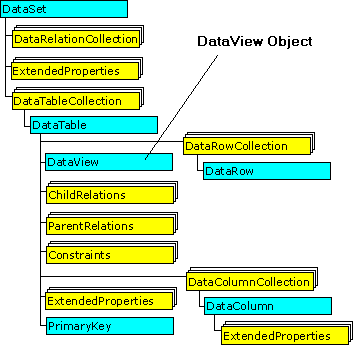